Part 1: Basic Data Structures In Go
Prerequisite: Basic Understanding of Golang syntax.
Array, Slice, Map, and Struct:
To understand Array and other data structure concepts, you need to know what is a 'Type' in Go.
Definition:
"A type determines a set of values together with operations and methods specific to those values. A type may be denoted by a type name if it has one, which must be followed by type arguments if the type is generic. A type may also be specified using a type literal, which composes a type from existing types."
Syntax:
type [identifier] [type literal]
Example:
type foo struct {
// statement
}
Or
type bar []string {
// statement
}
1. Array:
Definition:
An array is a numbered sequence of elements of a single type, called the element type. The number of elements is called the length of the array and is never negative.
The length of the array can be discovered using the built-in function len. Array types are always one-dimensional but may be composed to form multi-dimensional types.
Syntax:
[ArrayType] [identifier] [[ArrayLength]][ElementType]
Example:
package main
import "fmt"
func main() {
// Declares a variable x of type [10]int, an array of 10 integers.
var x [10]int
// Print the values of the array 'x'
fmt.Println("emp:", x)
// Print the type information of the variable 'x'
fmt.Printf("Type: %T\n", x)
// Print the length of the entire array.
fmt.Println("len:", len(x))
}
Output:
emp: [0 0 0 0 0 0 0 0 0 0]
Type: [10]int
len: 10
Go Playground: https://go.dev/play/p/cVrSTiQVuMT
Here we create an array that will hold exactly 10 ints. The type of elements and length are both parts of the array’s type. By default, an array is zero-valued, which for ints means 0s.
In Golang we don't use arrays due to their fixed size instead, we use slices.
2. Slice:
Definition:
A slice is a descriptor for a contiguous segment of an underlying array and provides access to a numbered sequence of elements from that array. A slice type denotes the set of all slices of arrays of its element type. The number of elements is called the length of the slice and is never negative. The value of an uninitialized slice is nil.
Unlike an array, a slice, on the other hand, is a dynamically-sized, flexible view into the elements of an array. In practice, slices are much more common than arrays.
Syntax:
var [identifier] [[]]
Example:
package main
import "fmt"
func main() {
// Create a slice 'x' of type int and initialize it with some values.
var x []int = []int{1, 4, 6, 8, 34, 54, 23, 12, 76, 23}
// Print all the values in the slice 'x'.
fmt.Println(x)
// Print the length of the silce.
fmt.Println(len(x))
// Print the type information of the variable 'x'
fmt.Printf("The type of x is: %T\n", x)
}
Output:
[1 4 6 8 34 54 23 12 76 23]
10
The type of x is: []int
Go Playground: https://go.dev/play/p/GUEoF-sBXyW

3. Map:
Definition:
A map is an unordered group of elements of one type, called the element type, indexed by a set of unique keys of another type, called the key type. The value of an uninitialized map is nil. A nil map has no keys, nor can keys be added.
A map maps keys to values.
The make function returns a map of the given type, initialized and ready for use.
Syntax
map[KeyType]ValueType
Example:
package main
import "fmt"
func main() {
// Create a map with a key of type string, and a value of type int.
m := make(map[string]int)
// Set the value of the key "foo" to 42.
m["foo"] = 42
// Print the value of the key "foo".
fmt.Println(m["foo"])
// Delete the key "foo".
delete(m, "foo")
// Print the value of the key "foo", which should now be zero.
fmt.Println(m["foo"])
}
Output:
42
0
Go Playground: https://go.dev/play/p/FJ9klmi69rC
4. Struct:
Definition:
A struct is a sequence of named elements, called fields, each of which has a name and a type. Field names may be specified explicitly (IdentifierList) or implicitly (EmbeddedField). Within a struct, non-blank field names must be unique.
Syntax
type StructType struct {
// Fields
}
Example:
package main
import "fmt"
func main() {
// This is a struct definition.
type FullName struct {
// FirstName defines the first name of a person.
FirstName string
// LastName defines the last name of a person.
LastName string
// Age defines the age of a person.
Age int
}
// Declares a struct variable named userName of type FullName and initializes it with the given values.
userName := FullName{
FirstName: "Alex",
LastName: "Jones",
Age: 34,
}
// Print the userName struct variable.
fmt.Println(userName)
}
Output:
{Alex Jones 34}
Go Playground: https://go.dev/play/p/LjSwFMfifsu
Conclusion
In conclusion, the basic data structures in Go are arrays, slices, maps, and structs. Each of these data structures has its own specific use cases and benefits. Arrays are best used for sequential data, slices for dynamic data, maps for key-value data, and structs for complex data.
Next article: Part 2: Binary Search using Go
Subscribe to my weekly newsletter for the latest insights on Golang, DevOps, Cloud-Native, Linux, Kubernetes, and more.
Follow me on Twitter!
Check out my GitHub for code snippets and more!
References:
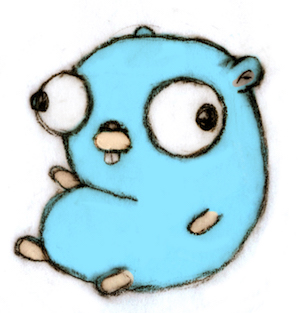